
Password show and hide onClick using vanilla javascript
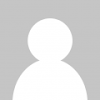
Hiding passwords visibility in forms helps protect from people looking over your shoulder and reading your password, bu…
Hiding passwords visibility in forms helps protect from people looking over your shoulder and reading your password, bu…
Today we are going to learn how to integrate google Language translator without showing powered by google using css. …
Download SkinFiner 2.0 Windows x64 with Crack Registration for Life Time SkinFiner 3.2 Crack is a natural photo impro…
Image preview is a great feature for user to check their image before upload whether the correct image is going to up…
Here's a generic way to do this that doesn't require you to use number_format or do string parsing: func…
This snippet will help you to get the name of your pc echo gethostbyaddr($_SERVER['REMOTE_ADDR']); echo …
How to replace multiple synonyms with one specific word using PHP $a = array( 'truck', 'vehicle',…
I am loading dynamically divs that have a .totalprice class. At the end, It will sum of the values from all of th…